Simple Migration Example
For most cases the conversion is straightforward. For example, consider the following MathScript function which plots sine and cosine waves.
This script in the MathScript node would look like:
t = 0:0.1:2*pi;
plot(t, sin(t), t, cos(t));
To convert this into MATLAB function and call it from LabVIEW:
- Create a .m file (say customPlot.m) and
- Create a function matching the name of the file, and copy the contents from the MathScript node as the function body:
function customPlot()
t = 0:0.1:2*pi;
plot(t, sin(t), t, cos(t));
end
- Call this function from LabVIEW by opening a MATLAB session. This is done via the Open MATLAB Session function.
- The session refnum is then passed into Call MATLAB function which is responsible for calling into .m functions.
- You must specify the path of the .m file as input to the node along with any other input parameters to the actual function itself.
- Finally, you close the session with a Close MATLAB Session function.
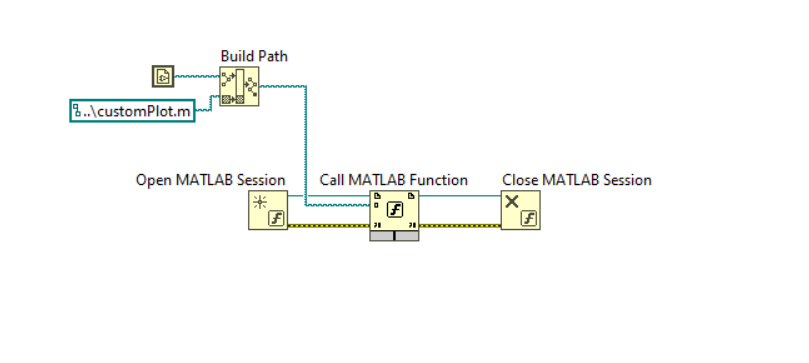
Complex Migration Example
Not all MathScript function names map directly to corresponding MATLAB function names. Consider the conversion of a more complex example.
The example below utilizes MathScript functions from the MathScript RT module. If you have the module, you can find the file in
<LabVIEW Directory>\examples\MathScript\MathScript Fractal.viThe MathScript Node for generating Mandelbrot Set looks like this:
The functions
timerstart,
timerstop,
linramp and
meshgrid2d are replaced with the corresponding MathWorks functions. The resulting MATLAB function would look like this:
function [xmult,ymult,time, W] = MandlebrotSet(xmax, xmin, ymax, ymin)
t = tic;
maxiter=50;
m=300;
xmult = (xmax-xmin)/m;
ymult = (ymax-ymin)/m;
x=linspace(xmin,xmax,m);
y=linspace(ymin,ymax,m);
[X,Y]=meshgrid(x,y);
Z=X+1i*Y;
c=Z;
for k=1:maxiter
Z=Z.^2+c;
end
W=288*real(exp(-abs(Z)))';
time=toc(t);
end
The first line indicates the function name; the input parameters are in the parenthesis after the function name and the outputs in the closing brackets. Here, we see that
timerstart and
timerstop are replaced by
tic and
toc;
linramp by
linespace and
meshgrid2d by
meshgrid.
When a MATLAB function, which returns multiple elements, is called from LabVIEW, we use a cluster with the same number of elements (as return types), for the return type. The types in the cluster should be ordered and each element should have the same name as the corresponding return type in the MATLAB function.
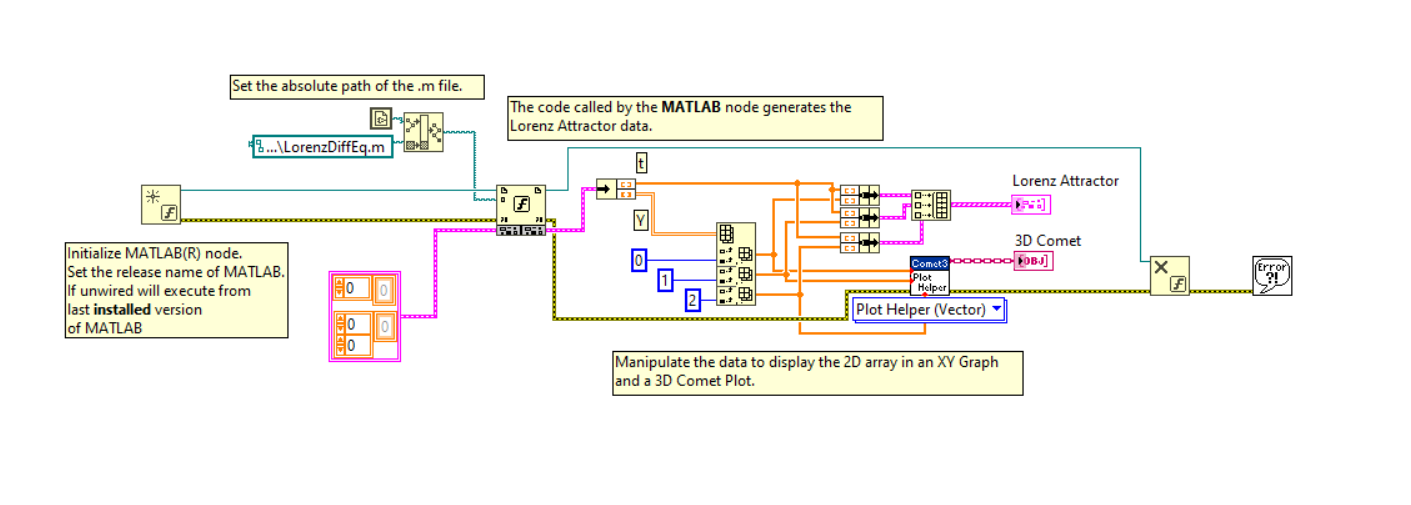
Note: Code above is from LabVIEW example
<LabVIEW 2021 Install Dir>\examples\Mathematics\Scripts and Formulas\MATLAB node - Lorenz Diff Eq.vi
MathScript Code Without Direct Mappings
Some MathScript functions may not have a direct mapping to MATLAB, but alternative for those can usually be found.
MathScript-Specific Functions
For instance, MathScript node will have access to more built-in functions and datatypes if you have LabVIEW Control Design and Simulation (CD & Sim) module installed.
One such example is the function
ackermann. With CD & Sim installed, this function can readily be invoked in LabVIEW MathScript code as a built-in function. In base MATLAB there is no built-in equivalent, but through the community’s
File Exchange page, you can find suitable alternatives like this
one.
MATLAB-Specific Functions
For some built-in functions you would also need to install additional MathWorks products on top of MATLAB.
For example, the
ss function is not present in the base MATLAB and would require additional addons to be installed. Using the function in MATLAB console will prompt the missing addon which is required for the function execution.
MATLAB® is a registered trademark of The MathWorks, Inc.