Visual Basic .NET and Visual C#
Many IVI and VXI plug&play drivers include C DLLs, which provide functions that communicate with a particular instrument. To use an IVI or VXI plug&play driver with a C DLL in a Measurement Studio .NET application, use the .NET Instrument Driver wizard to create .NET entry points to the DLL functions you need to call from your application. Measurement Studio 7.1 and later includes the Measurement Studio Instrument Driver .NET Wizard.
The .NET Instrument Driver wizard generates a .NET wrapper class for calling into IVI, VXI plug&play, and legacy instrument drivers based on the instrument driver function panel, header file, and an optional .sub file for IVI drivers. The wizard can generate both Visual C# and Visual Basic .NET source code.
NI has already generated wrappers for the Modular Instruments drivers. Information on these wrappers can be found within the NI's .NET Support documentation. You should also be aware that for High Speed Digitizers there is a native NI-SCOPE .Net driver. More information on this driver is available in the following article NI-SCOPE .NET Resources.
Generating an instrument driver wrapper class with the .NET Instrument Driver wizard
-
Open the Visual Basic .NET or Visual C# project in which you want to generate an instrument driver wrapper class.
-
Select Project»Add New Item to launch the Add New Item dialog box.
-
In the Categories pane, select Measurement Studio.
-
In the Templates pane, select Instrument Driver.
-
Specify a name for the .NET instrument driver and click Open. The name you choose for the file becomes the name of the source file.
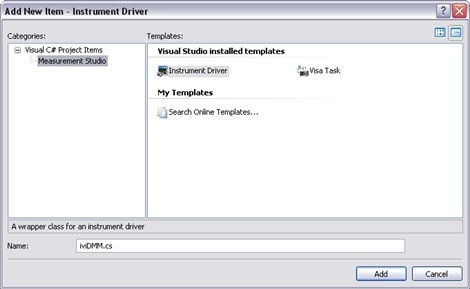
Figure 1. Add New Item dialog
-
In the New .NET Instrument Driver dialog box, select which instrument driver you want to create by browsing to the .fp filename. Click Next.
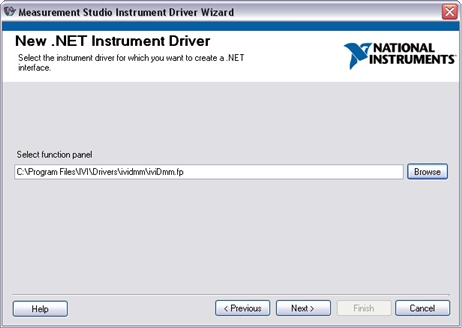
Figure 2. New .NET Instrument Driver dialog
-
Configure the code generation options. Default values are given based on information from the instrument driver .fp file.
-
Click Finish.
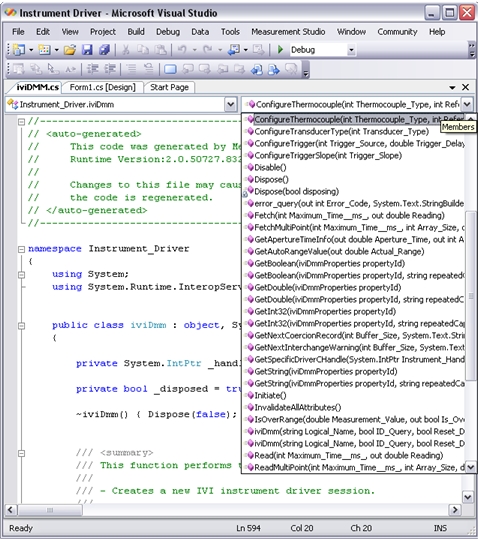
Figure 3. Generated instrument driver wrapper class
After you complete these steps, a new instrument driver wrapper class is added to the project and opened in the source code editor. Now you can edit and compile this file the same as any other source file in your project.
Inspecting an instrument driver wrapper class
After the .NET Instrument Driver wizard finishes, inspect the top of the file for any comments that begin with TODO. These comments indicate problems the wizard encountered while generating the instrument driver wrapper class, such as duplicate entries in the .fp or .sub file or missing constant values in the instrument driver header file.
If you chose to generate documentation comments, the comments precede each method in the instrument driver wrapper class. This help content also appears in Intellisense when you use the class. Because the wizard imports documentation comments directly from the function panel file, the documentation refers to elements in the driver's C API. For example, the documentation comments might refer to a function named niScope_init rather than to the class constructor, or the documentation might refer to an attribute named NISCOPE_ATTR_RANGE_CHECK rather than the NiScopeProperties.RangeCheck constant.
Calling an instrument driver wrapper class
The following example is a typical example of how you use an instrument driver wrapper class. Note that this example uses the pre-generated instrument driver wrapper class for the NI's NI-SCOPE instrument driver. The example code will not run unless NI-SCOPE is installed and an NI-SCOPE device is present in the system. You can download the NI-SCOPE instrument driver wrapper from NI-SCOPE .NET Resources.
[C#] using (niScope scope = new niScope("myDAQmxDevice", true, true)) { double[] waveform = new double[1000]; niScopeWfmInfo[] waveformInfo = new niScopeWfmInfo[1];
scope.ConfigureChannel("0", 10.0, 0.0, niScopeConstants.Ac, 1.0, true); scope.SetString(niScopeProperties.TriggerSource, "0"); scope.Read("0", 0.0, waveform.Length, waveform, waveformInfo); }
[VB.NET] Using scope = New niScope("myDAQmxDevice", True, True) Dim waveform(999) As Double Dim waveformInfo(0) As niScopeWfmInfo
scope.ConfigureChannel("0", 10.0, 0.0, niScopeConstants.Ac, 1.0, True) scope.SetString(niScopeProperties.TriggerSource, "0") scope.Read("0", 0.0, waveform.Length, waveform, waveformInfo)
End Using
|
If you do not have Measurement Studio and want to use an instrument driver DLL in a Visual Basic .NET or Visual C# project, you must use the DllImportAttribute class in the project. Refer to the Microsoft .NET Framework help for information about the DllImport attribute. For information about the functions in each instrument driver, refer to the corresponding help file installed with each driver.
Visual Basic 6
Complete the following steps to reference an instrument driver DLL in a Visual Basic 6 project.
-
Select Project»References.
-
Click Browse in the References dialog box and navigate to the location of the instrument driver DLL. Most National Instruments' instrument driver DLLs are installed by default in c:\Program Files\IVI\Bin directory.
-
Select the instrument driver DLL to add to the project.
-
After you add a reference to the project for an instrument driver DLL, use the Object Browser (View»Object Browser) to view the functions and parameters available in each instrument driver.
-
Now you can use any of the functions in the instrument driver. For information about the functions in each instrument driver, refer to the corresponding help file installed with each driver.