Depending on which version of LabVIEW you are using, choose from the following methods to stop multiple parallel While Loops in LabVIEW using one stop button.
Note: The methods listed below are not an exhaustive presentation of the methods to stop parallel While Loops. These methods are the most common methods.
Channel Wires
If you are using LabVIEW 2016, you can use channel wires to stop multiple parallel While Loops with one stop button. This is possible because channel wires are asynchronous wires that connect two parallel While Loops without forcing an execution order. Hence, no data dependency between the two parallel While Loops.
Note: Channel wires are supported by the LabVIEW Real-Time Module but not the LabVIEW FPGA Module.
The following block diagram demonstrates stopping parallel While Loops using a Tag channel:
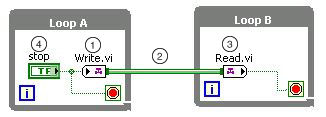
The following list describes important details about the previous block diagram:
- Loop A contains a Tag writer endpoint that writes values to a Tag channel.
- A channel wire connects the channel output of the Tag writer endpoint to a Tag reader endpoint.
- Loop B contains a Tag reader endpoint that reads values from a Tag channel.
- The stop button wired to the element parameter of the writer endpoint in Loop A communicates with Loop B. If you click the stop button in Loop A, Loop B also stops.
The following block diagram demonstrates stopping parallel While Loops using a Stream channel:
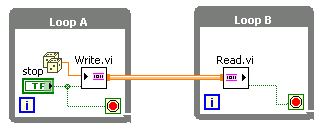
When using Stream channels, the stop button wired to the last element parameter of the writer endpoint in Loop A communicates with Loop B.
The Tag channel and the Stream channel have major differences when used to stop parallel While Loops.
- Using a Tag channel to stop parallel While Loops causes all loops to stop as soon as possible because the stop button value from Loop A communicates with Loop B directly. Using a Stream channel to stop parallel While Loops may cause Loop B to stop several iterations later than Loop A because the stop button value from Loop A communicates with Loop B through the last element?parameter, which means Loop A stops as soon as it has written the last element. However, Loop B continues to read the remaining elements in the channel and will not stop until it has read the last element. The Stream channel ensures that all data elements communicated through the channel gets processed before Loop B stops.
- The Tag channel allows you to stop two or more parallel While Loops because the Tag channel can be forked into multiple writers and readers. The Stream channel allows you to stop only two parallel While Loops because the Stream channel allows only one writer and one reader.
The following block diagram demonstrates using the
Tag channel to stop three parallel While Loops.
Caveat
You cannot extend the previous Tag architecture to have multiple stop buttons. However, you can add one or more stop buttons using the Share Stop Signal VI located in labview\vi.lib\ChannelSupport
, which allows you to stop multiple parallel While Loops with any one of the stop buttons.
The following VI snippet demonstrates stopping multiple parallel While Loops using either of the two stop buttons. To add more stop buttons, follow the same architecture.

Local Variables
You can stop multiple parallel While Loops with one stop button by passing the Boolean stop button value from your first loop's control to all of the other loops using a local variable for each additional loop. The following block diagram demonstrates stopping two parallel While Loops using a local variable.
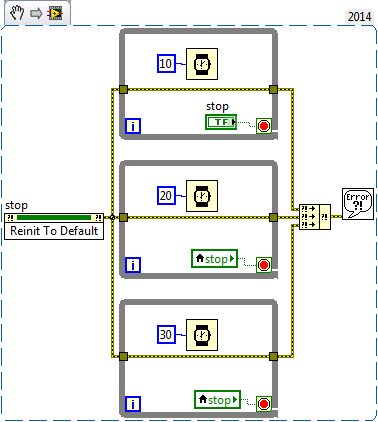
The following list describes important details about the previous block diagram:
- The top loop contains a stop control. Configure the mechanical action of the stop control by right-clicking the stop control on the front panel and selecting Mechanical Action»Switch When Pressed. This allows for all of the loops to read the stop boolean, where as latch when pressed will reset to true when the value is read leaving some loops still running.
- The bottom two loops contains a local variable of the stop control. The local variable is created by right-clicking the stop control and selecting Create»Local Variable. Configure the local variable to read the value by right-clicking the local variable and selecting Change to Read
- It is important to note the Reinit To Default invoke node that is implicitly referencing the stop button, this resets the value to true at the beginning of the next run.
- All of these loops contain a Wait ms to highlight that all of the loops stop, regardless of their different run times.
To stop more than three parallel While Loops, follow the same architecture by adding more local variables to read from the initial stop control.
Property Nodes
You can use a property node to pass the Boolean stop button value from your first loop's control to all of the other loops. The following block diagram demonstrates stopping two parallel While Loops using a property node.
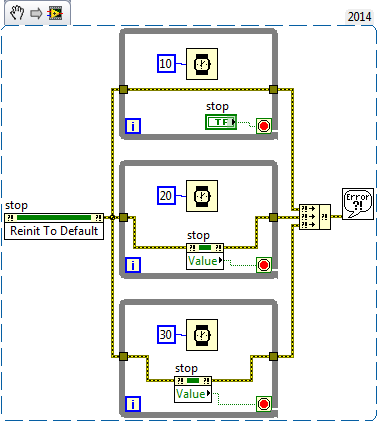
The following list describes important details about the previous block diagram:
- The top loop contains a stop control. Configure the mechanical action of the stop control by right-clicking the stop control on the front panel and selecting Mechanical Action»Switch When Pressed. This allows for all of the loops to read the stop boolean, where as latch when pressed will reset to true when the value is read leaving some loops still running.
- The bottom loop contains a property node of the stop control. The property node is created by right-clicking the stop control and selecting Create»Property Node»Value. Configure the Value property node to read the value by right-clicking the Value property node and selecting Change to Read.
- It is important to note the Reinit To Default invoke node that is implicitly referencing the stop button, this resets the value to true at the beginning of the next run.
- All of these loops contain a Wait ms to highlight that all of the loops stop, regardless of their different run times.
To stop more than two parallel While Loops, follow the same architecture by adding more Value property nodes to read from the initial stop control.
For more information about when to use a local variable versus a property node, refer to Control/Indicator, Local Variable, and Value Property Node Differences.
Notifiers
For applications that involve complex behavior, notifiers can be used to stop multiple loops running in parallel. The notifier functions are accessible from the Programming»Synchronization»Notifier Operations palette.
Note: The use of notifiers scales easily to multiple threads, but does not always integrate well with existing program architectures.
The following VI snippet demonstrates one way notifiers can be used to stop multiple While Loops.
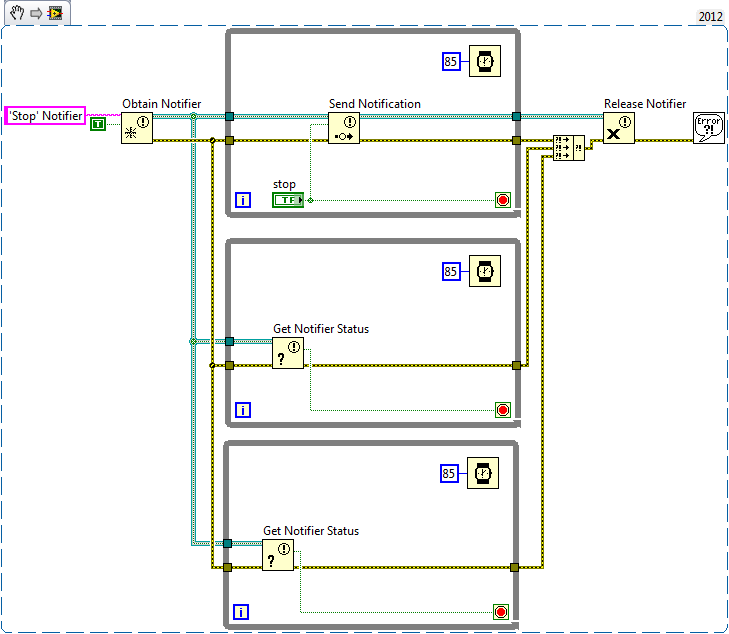
The following list describes important details about the previous VI snippet:
Queues
You can use queues to stop multiple loops running in parallel for applications that involve more complex behavior. The queue functions are accessible from the Programming»Synchronization»Queue Operations palette.
Note: Many larger applications already have a queue system in place to exchange data between parallel loops. In these setups, the existing queue may be modified or enhanced to include a stop instruction for parallel loops.
The following VI snippet demonstrates using queues to stop multiple While Loops:
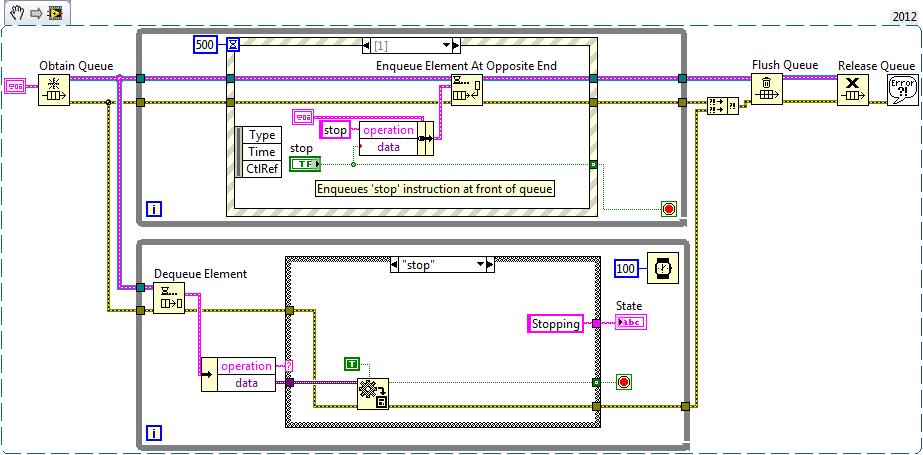
Some applications using a Master-Slave architecture release the queue before the slave threads exit. The error thrown from dequeueing from a destroyed reference is then used to stop the slave loops. In lieu of relying on an error condition to stop the slave loops, the previous VI snippet has a discrete stop instruction that is passed on to the slave loop from the master loop.