There is a third-party wrapper called
pyVirtualBench that provides a Python interface to the VirtualBench hardware. It does so by wrapping the VirtualBench's driver's C application programming interface.
Note: This wrapper is third-party software and not directly supported by National Instruments.
Follow the steps below to test the connection to your VirtualBench from Python.
- Make sure to meet these general requirements:
- Verify the connection between your computer and your powered-on VirtualBench hardware using either NI MAX or the NI VirtualBench Application.
- Find your VirtualBench's name using either NI MAX or the NI VirtualBench Application: Go to menu File ยป About
NI MAX:
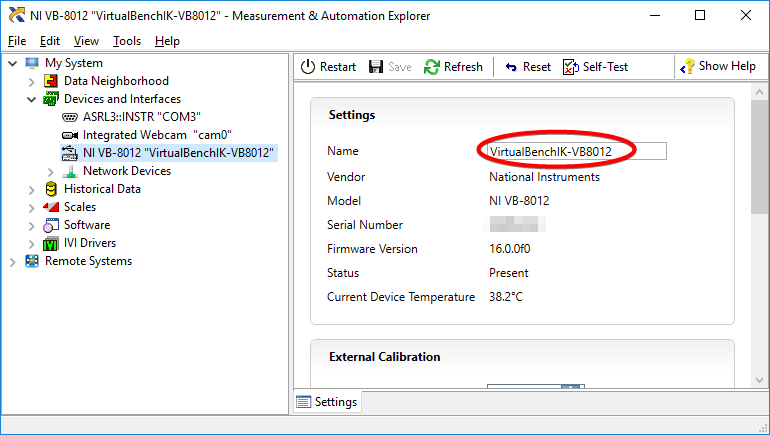
NI VirtualBench Application:
- Adapt line 5 of the following Python script to reflect your hardware's name:
#!/usr/bin/env python
from pyvirtualbench import PyVirtualBench, DmmFunction, PyVirtualBenchException
# Change the next line before executing!
virtualbench = PyVirtualBench("VirtualBenchIK-VB8012")
# Device ID as found in NI VirtualBench Application in File > About
# The device's ID can be changed via the NI VirtualBench Application, File > Preferences
try:
dmm = virtualbench.acquire_digital_multimeter()
dmm.configure_measurement(DmmFunction.DC_VOLTS, True, 10)
myData = dmm.read()
print(myData)
dmm.release()
except PyVirtualBenchException as e:
print("Error/Warning %d occured: %s" %(e.status, e))
finally:
virtualbench.release()
- Depending on the setup of your operating system's environment variable path and of your Python environment, you might have to add a reference to the new package's location to your code. If this is the case, insert a sys.path.append statement as second line into the script above, replacing the placeholder with the path to the .py package file:
#!/usr/bin/env python
sys.path.append(os.path.abspath("<absolute path to pyvirtualbench location>")
from pyvirtualbench import PyVirtualBench, DmmFunction, PyVirtualBenchException
[...]
- Execute the script. It connects to the VirtualBench hardware, sets up the Digital Multimeter, reads one voltage value, and outputs the value on the screen. Connect probes to the "HI" and "LOW" connectors on the VirtualBench's frontpanel to measure real values.