Solution
Benchmarking sections of code
To measure elapsed time in a piece of code, the best way is to use a Flat Sequence Structure in conjunction with a time count function or VI. Because the Flat Sequence Structure forces the code to run sequentially, you can use measure the time before and after your code runs. Subtract the time measurement before from the time measurement after to get elapsed time (delta t).
The time count function or VI you should use depends on your use case.
Tick Count (ms)
For simple code measurements, use the
Tick Count (ms) function.
- This is a good timing structure if your code runs on the order of milliseconds even up to days.
- The code being measured in the snippet below is a Wait (ms) function. Replace the Wait (ms) function with your own code to benchmark it
- The snippet below will run once and give you a single timing comparison. If you would like to run code multiple times to get an average runtime, you can use the Measure Average Code Execution Time Using LabVIEW example code which iterates code and outputs the average execution time.
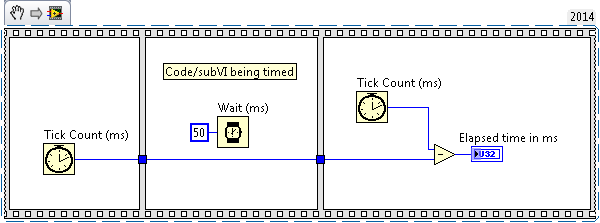
Get Date/Time in Seconds
If your code needs to run on the order of months, use the
Get Date/Time in Seconds function. Again, the code being measured in the snippet below is a Wait (ms) function. Replace the Wait (ms) function with your own code to benchmark it.
Tick Count Express VI (Real-Time)
If you are benchmarking code on a Real-Time target, you can use the
Tick Count Express VI and configure it to show the elapsed time in ticks so you will have a more precise result.
- Note that you can use this function on a Windows or other non-deterministic OS, but it will only have precision up to the millisecond.
- Again, the code being measured in the snippet below is a Wait (ms) function. Replace the Wait (ms) function with your own code to benchmark it
Benchmarking Loops
You can benchmark how long it takes to iterate through a while loop (or a for loop) by using the Tick Count (ms) to take the current time in milliseconds and subtract it from the time during the previous iteration, which is saved in the shift register.
You can code an alternate version that uses a Feedback Note instead of Shift Registers. You just need to drop the following code inside your while loop and get the Iteration time (ms) without any modification to your original code.
Note: The images above are LabVIEW code snippets, which include LabVIEW code that you can reuse in your project. To use a snippet, right-click the image, save it to your computer, and drag the file onto your LabVIEW diagram.