Additional Information
Consider the following code snippet:
namespace TestDLL
{
public class Class2
{
public int number1 = 2;
public int number2 = 4;
public int number3 = 99;
}
public class Class1
{
public void square(ref int number)
{
number *= number;
}
public void add2(ref Class2 newclass)
{
newclass.number3 = newclass.number1 + newclass.number2;
}
public int add(int number 1, int number2)
{
return number1 + number2;
}
}
}
Using the ref keyword in the function prototype forces LabVIEW to provide both input and output nodes when calling the object method. As a result, the corresponding code for calling the .NET DLL in LabVIEW is a such.
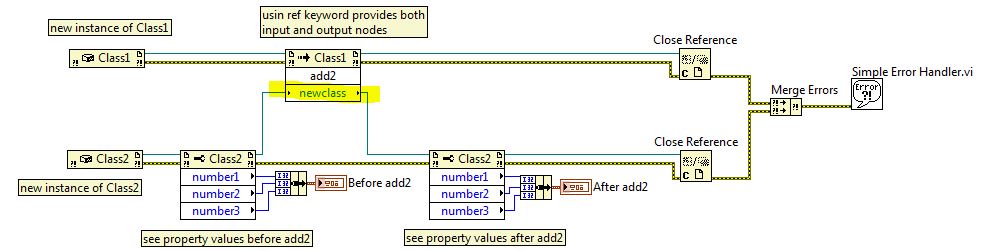
The results are as follows, with the expected output.
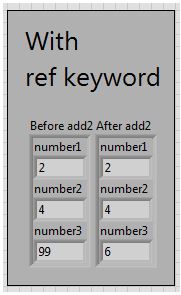
Omitting the ref keyword with the following code snippet:
namespace TestDLL
{
public class Class2
{
public int number1 = 2;
public int number2 = 4;
public int number3 = 99;
}
public class Class1
{
public void square(ref int number)
{
number *= number;
}
public void add2(Class2 newclass)
{
newclass.number3 = newclass.number1 + newclass.number2;
}
public int add(int number 1, int number2)
{
return number1 + number2;
}
}
}
The corresponding code would be as such:

Running the program yields the same results.